Private static and instance members in mootools classes
You can also be interested in:
Let's see two patterns that may be used to have private members in mootools classes.
Private static members
Here is quite simple, it's enough to use a single execution function and js closures and provide the setter and getter methods to change and retrieve the member's values.
var myclass = (function() {
var _name = null,
_age = null;
return new Class({
initialize: function() {
// do some stuffs
},
setName: function(name) {
// do some checks here
_name = name;
},
setAge: function(age) {
// do some checks here
_age = age.toInt();
},
getName: function() {
return _name;
},
getAge: function() {
return _age;
}
});
}());
var myinstance = new myclass();
console.log(myinstance.name); // undefined
myinstance.setName('jack');
console.log(myinstance.name); // undefined
console.log(myinstance.getName()); // jack
So what happens here is that the two variables
_name
and
_age
are in the scope of the single execution function, and so not accessible from outside. But their value is retained by the returned object which yes can access them since it is inside the single execution function, so it may set and get the variables values and make them accessible through these getter and setter methods.
But what is important to notice here is that such private members are class members or static members, since are shared by all class instances!
Try yourself:
var myinstance1 = new myclass();
var myinstance2 = new myclass();
myinstance1.setName('jack');
console.log(myinstance1.getName()); // jack
myinstance2.setName('jeff');
console.log(myinstance2.getName()); // jeff
console.log(myinstance1.getName()); // jeff !!!!
So, if you want instance members you have to add some tricks
Private members
Here the trick is to make our private variable properties of an object inside a 'class id' property which keep the reference to the instance, let's see the code
var ids = 0;
var myclass = (function() {
var _private = {};
return new Class({
initialize: function() {
// do some stuffs
this.id = ids++;
_private[this.id] = {};
},
setName: function(name) {
// do some checks here
_private[this.id].name = name;
},
setAge: function(age) {
// do some checks here
_private[this.id].age = age.toInt();
},
getName: function() {
return _private[this.id].name;
},
getAge: function() {
return _private[this.id].age;
}
});
}());
var myinstance1 = new myclass();
var myinstance2 = new myclass();
myinstance1.setName('jack');
console.log(myinstance.getName()); // jack
myinstance2.setName('jeff');
console.log(myinstance2.getName()); // jeff
console.log(myinstance1.getName()); //jack
Every instance creates a new property of the object
_private
and stores its private members inside it, clearly is very important here to generate a unique class id.
The next time we'll see how to implement protected properties in mootools class inheritance, using a similar pattern.
Your Smartwatch Loves Tasker!
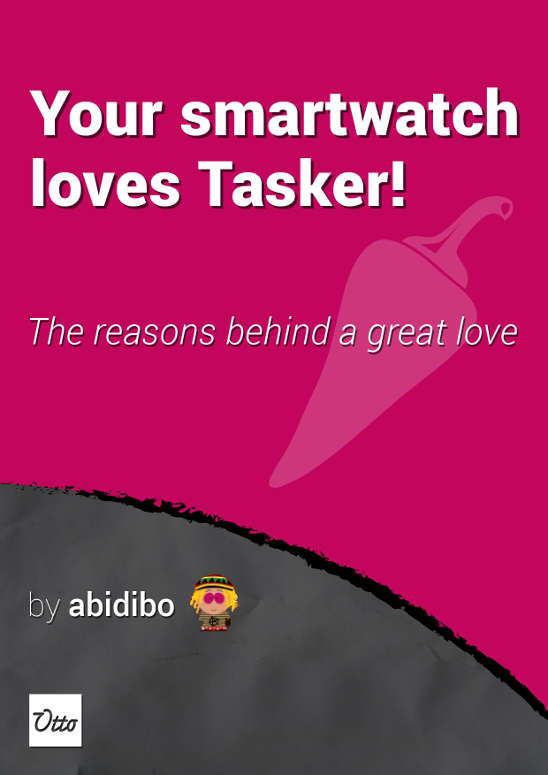
Your Smartwatch Loves Tasker!
Featured
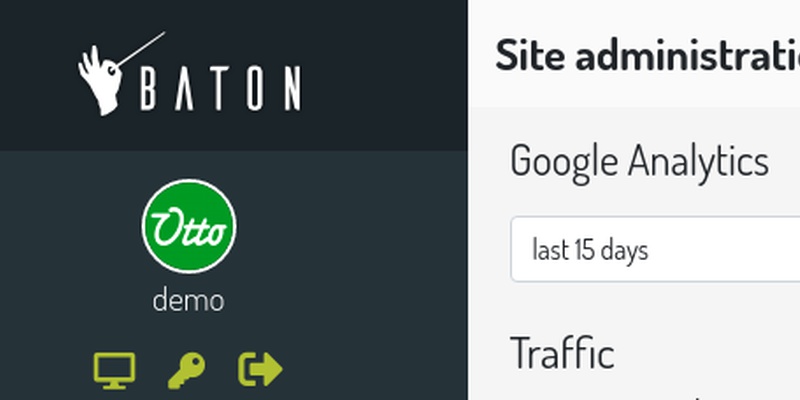
Django admin and bootstrap 5
Bootstrap 5 has come, currently in beta release, and seems already very stable.
So the question is: are you looking for ...
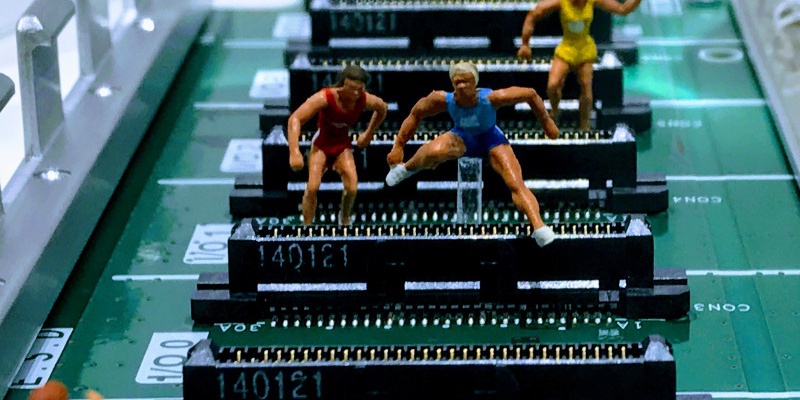
About code optimization, learn from exercises
Let's see an example of exercise you can face during a job interview, and let's use it to understand some ...
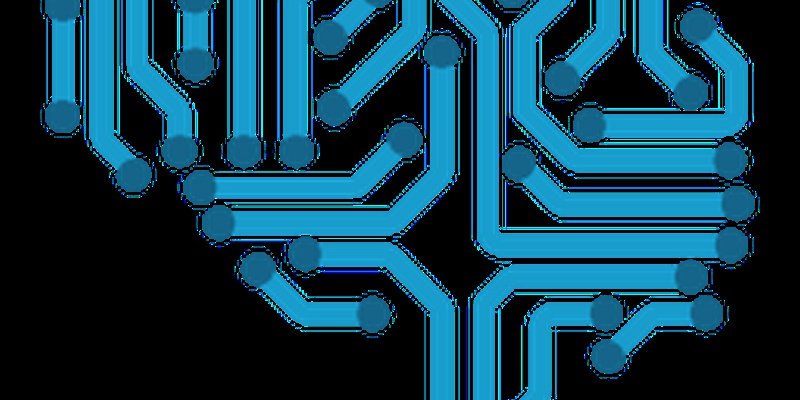
Notes on the Pearson correlation coefficient
The Pearson correlation coefficient is a measure of the linear correlation between two variables X and Y. It has a ...
Archive
- 2021
- 2020
- 2019
- 2018
- 2017
- Nov
- Oct
- Aug
- Jun
- Mar
- Feb
- 2016
- Oct
- Jun
- May
- Apr
- Mar
- Feb
- Jan
- 2015
- Nov
- Oct
- Aug
- Apr
- Mar
- Feb
- Jan
- 2014
- Sep
- Jul
- May
- Apr
- Mar
- Feb
- Jan
- 2013
- Nov
- Oct
- Sep
- Aug
- Jul
- Jun
- May
- Apr
- Mar
- Feb
- Jan
- 2012
- Dec
- Nov
- Oct
- Aug
- Jul
- Jun
- May
- Apr
- Jan
- 2011
- Dec
- Nov
- Oct
- Sep
- Aug
- Jul
- Jun
- May